Yeah, @JustPete - If you stared at this all day, I feel for ya and want to give you two related code examples. Try out each of these two patterns on your board and see if you understand what’s going on.
Populating an array's values manually
Let’s just work with the first 5 of your pixels for this one.
export var data = array(5) // Set up a 5-bin container
// Fill it with some data
data[0] = 0
data[1] = 1
data[2] = 0
data[3] = .5
data[4] = 1
// Notice there's no beforeRender(). Doni't need it this time.
export function render(index) {
if (index >= 5) { // All pixels after the fifth are dark
hsv(0, 0, 0)
} else {
h = data[index] ? 0.0 : 0.66 // Red if this bin is nonzero, Blue if it's zero
s = 1
v = data[index] ? 1 : .3 // Full brightness if this bin is nonzero, 30% otherwise
hsv(h, s, v)
}
}
Output:
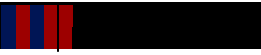
For loop
If you follow that, here’s how we can use the for loop to populate an array. You don’t really need an array to make this pattern, but let’s try anyway. Make the perfect squares (0, 1, 4, 9, 16, 25, 36, 49, etc) bright.

var brightnesses = array(pixelCount)
// Loop through all the bins, setting the brightness for each pixel
for (i = 0; i < pixelCount; i++) {
// If there's no decimal part to the square root,
// it's a perfect square (0,1,4,9,16,25,etc);
// set it's brightness to be full
if (frac(sqrt(i)) == 0) {
brightnesses[i] = 1
} else {
brightnesses[i] = .1
}
}
export function render(index) {
h = index/pixelCount // Rainbow
s = 1
v = brightnesses[index]
hsv(h, s, v)
}
Hope this helps demystify arrays.